All
- Hello world in python
- How to add two numbers in python
- If else statement python
- Lists tuples and dictionaries
- Loops in python
- Python Functions
- How To Reverse Number In Python
- How to create voice from words and How to play music in Python
- How to Sort Dictionary using keys or values python
- Python Lambda
- Python map() filter() and reduce() functions
- What does if __name__ == "__main__": do?
What does if __name__ == "__main__":
do?
__name__
is a global variable (in Python, global actually means on the module level) that exists in all namespaces. It is typically the module's name (as a str
type).
As the only special case, however, in whatever Python process you run, as in mycode.py:
python any_snippet.py
the otherwise anonymous global namespace is assigned the value of '__main__'
to its __name__
.
Thus, including the final lines
if __name__ == '__main__':
main()
- at the end of your mycode.py script,
- when it is the primary, entry-point module that is run by a Python process,
will cause your script's uniquely defined main
function to run.
Another benefit of using this construct: you can also import your code as a module in another script and then run the main function if and when your program decides:
import mycode
#Your code goes here
mycode.main()
Subscribe to my youtube channel
All
May 7, 2021, 7:20 a.m.
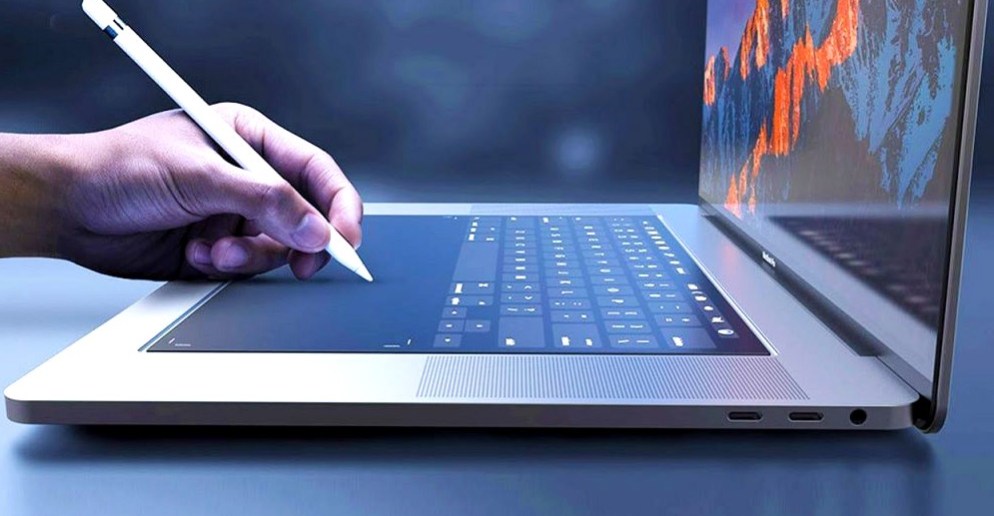
May 23, 2021, 12:19 p.m.
June 21, 2021, 3:08 p.m.
Aug. 26, 2021, 10:22 a.m.