All
- Hello world in python
- How to add two numbers in python
- If else statement python
- Lists tuples and dictionaries
- Loops in python
- Python Functions
- How To Reverse Number In Python
- How to create voice from words and How to play music in Python
- How to Sort Dictionary using keys or values python
- Python Lambda
- Python map() filter() and reduce() functions
- What does if __name__ == "__main__": do?
Functions
Functions are of two types, either they will return some results or they just do processing inside.
Lets define a simple function that take two arguments and return addition of those two variables.
def main(a,b):
return a+b
result = main(2,3)
print("Result of 2 passed arguments is : ",result)
In the same way lets create simple python function that will return square of argument passed in function
def main(a):
return a**2
print(main(3))
You can also pass as many arguments in function as you want, for that use *args as an argument and list of arguments will be created in args variable and you can iterate through it.
def main(*args):
for i in args:
print(i)
print(main(3,4,"Usman","Alex","Maria"))
and it will print out :
3
4
Usman
Alex
Maria
Subscribe to my youtube channel
All
May 7, 2021, 7:20 a.m.
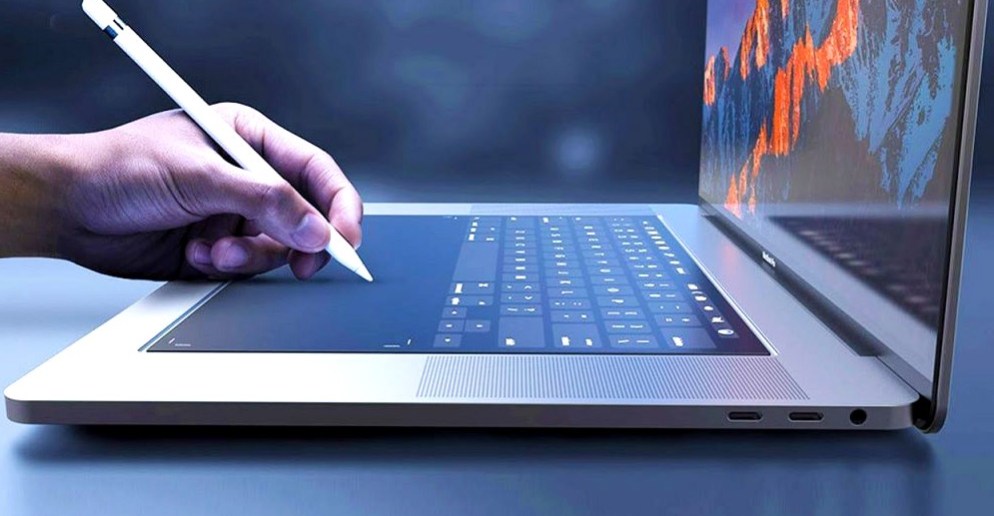
May 23, 2021, 12:19 p.m.
June 21, 2021, 3:08 p.m.
Aug. 26, 2021, 10:22 a.m.