All
- Hello world in python
- How to add two numbers in python
- If else statement python
- Lists tuples and dictionaries
- Loops in python
- Python Functions
- How To Reverse Number In Python
- How to create voice from words and How to play music in Python
- How to Sort Dictionary using keys or values python
- Python Lambda
- Python map() filter() and reduce() functions
- What does if __name__ == "__main__": do?
Lists tuples and dictionaries
Lists
List is a non-homogeneous data structure which stores the elements in single row and multiple rows and columns.
Benifit of list over simple python variable is that python you can store multiple type of values in a single variable.
Some important things to remember about lists are :
1. List can be represented by [ ]
2. List allows duplicate elements
3. List can use nested among all
4. Example: [1, 2, 3, 4, 5]
5. List is mutable i.e we can make any changes in list.
Following code will create a simple empty python list
lst = []
to access elements,type name of element and then index (Index start from 0)
e.g Assume
lst = ["Alex",12,13,"usman",15,18]
Now lets say you want to grab alex so you can use
print(lst[0])
If you want to grab multiple values you can use slicing.
For example if you want to grab Alex,12 and 13 you can write
print(lst[0:3])
and it will output : Alex,12,13
Below are some list methods which you can use
Method | Description |
---|---|
append() | Adds an element at the end of the list |
clear() | Removes all the elements from the list |
copy() | Returns a copy of the list |
count() | Returns the number of elements with the specified value |
extend() | Add the elements of a list (or any iterable), to the end of the current list |
index() | Returns the index of the first element with the specified value |
insert() | Adds an element at the specified position |
pop() | Removes the element at the specified position |
remove() | Removes the first item with the specified value |
reverse() | Reverses the order of the list |
sort() | Sorts the list |
Tuples
Tuple is also a non-homogeneous data structure which stores single row and multiple rows and columns
1. Tuple can be represented by ( )
2. Tuple allows duplicate elements
3. Tuple can use nested among all
4. Example: (1, 2, 3, 4, 5)
5. Tuple can be created using tuple() function.
Tuple is immutable i.e we can not make any changes in tuple
Following code will create an empty Tuple
Picking elements on basis of index and doing slicing is same as lists which is defined above.
Dictionaries
Dictionary is also a non-homogeneous data structure which stores key value pairs
1. Dictionary can be represented by { }
2. Dictionary will not allow duplicate elements but keys are not duplicated
3. Dictonary can use nested among all
4. Example: {1 : "usman", 2 : "ali"}
5. Dictonary can be created using dict() function.
6. Dictionary is mutable. But Keys are not duplicated.
7. Dictionary is ordered
8. Creating an empty dictionary
9. dictionary={}
Examples
# Dictonary d = {} # Adding the key value pair d[1] = "One" d[2] = "Two" print("Dictonary", d) # Removing key-value pair del d[2] print("Dictonary", d) #output ''' { 1 : "One" } '''
To get keys of dictionaries use dict.keys(), to get values of dictionary use dict.values()
Subscribe to my youtube channel
All
May 7, 2021, 7:20 a.m.
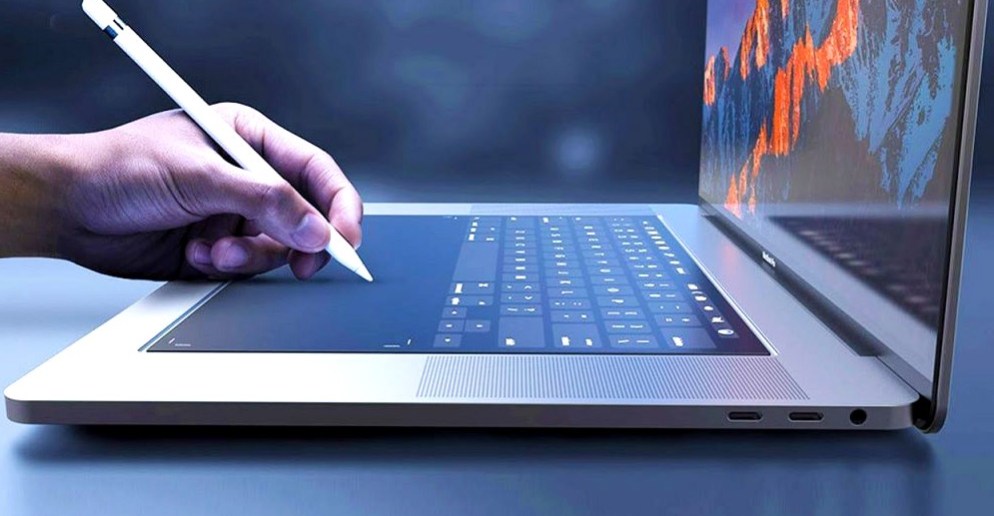
May 23, 2021, 12:19 p.m.
June 21, 2021, 3:08 p.m.
Aug. 26, 2021, 10:22 a.m.