All
- Hello world in python
- How to add two numbers in python
- If else statement python
- Lists tuples and dictionaries
- Loops in python
- Python Functions
- How To Reverse Number In Python
- How to create voice from words and How to play music in Python
- How to Sort Dictionary using keys or values python
- Python Lambda
- Python map() filter() and reduce() functions
- What does if __name__ == "__main__": do?
Python Map, Filter & Reduce pythonocean python ocean
The map() Function
The map()
function iterates through all items in the given iterable i.e list etc and executes the function we passed as an argument.
The syntax is of map() function is following:
map(function, iterable(s))
Example of map is following
def filter_even_numbers(s): if s%2==0: return s nums = [2,3,4,5,6] map_object = map(filter_even_numbers, nums) print(list(map_object)) ## It will output an array with values : [2,None,4,None,6]
Above code will filter out even numbers and print them out.
The Filter() Function
Similar to map() function, filter()
takes a function object and creates a new list.
As the name suggests, filter()
create a new list that contains only elements that satisfy a certain condition.
Syntax is following
filter(function, iterable(s))
def filter_even_numbers(s): if s%2==0: return s nums = [2,3,4,5,6] map_object = filter(filter_even_numbers, nums) list(map_object) ## It will output an array with values : [2,4,6]
The Reduce Function()
Reduce ()
works differently from map () and filter (). It does not return any new list based on function and what we have passed is repetitive. Instead, it returns a single value.
In addition, reduce () in Python 3 no longer has the built-in function, and it can be found in the Functools module.
from functools import reduce def add(x, y): return x + y list = [3, 8, 10] print(reduce(add, list)) ## It will output sum of elements of list that is 21
Subscribe to my youtube channel
All
May 7, 2021, 7:20 a.m.
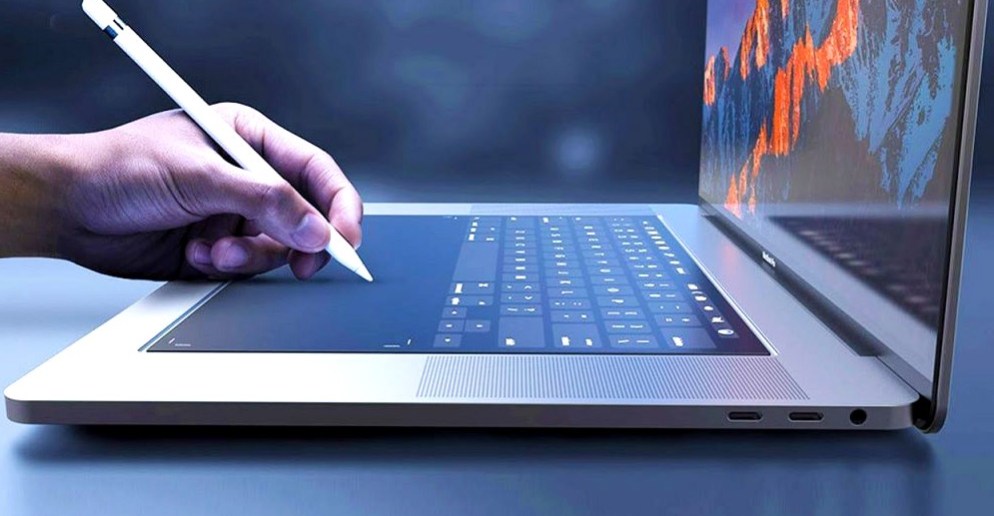
May 23, 2021, 12:19 p.m.
June 21, 2021, 3:08 p.m.
Aug. 26, 2021, 10:22 a.m.