All
- Hello world in python
- How to add two numbers in python
- If else statement python
- Lists tuples and dictionaries
- Loops in python
- Python Functions
- How To Reverse Number In Python
- How to create voice from words and How to play music in Python
- How to Sort Dictionary using keys or values python
- Python Lambda
- Python map() filter() and reduce() functions
- What does if __name__ == "__main__": do?
How to Sort Dictionary using keys or values in python
As there is no such method as .sort() or sorted() to sort dictionary so lets apply some logic,
Below code can be used to sort dictionary on basis of keys
dictionary = {1:2 , 4:3 , 3:4 , 0:0 , 2:1}
print({x: y for x, y in sorted(dictionary.items(), key=lambda item: item[0])})
and to sort dictionary on basis of values, copy following lines of code into your script
dictionary = {1:2 , 4:3 , 3:4 , 0:0 , 2:1}
print({x: y for x, y in sorted(dictionary.items(), key=lambda item: item[1])})
Subscribe to my youtube channel
All
What is python programming and why you should learn it in 2021
May 7, 2021, 7:20 a.m.
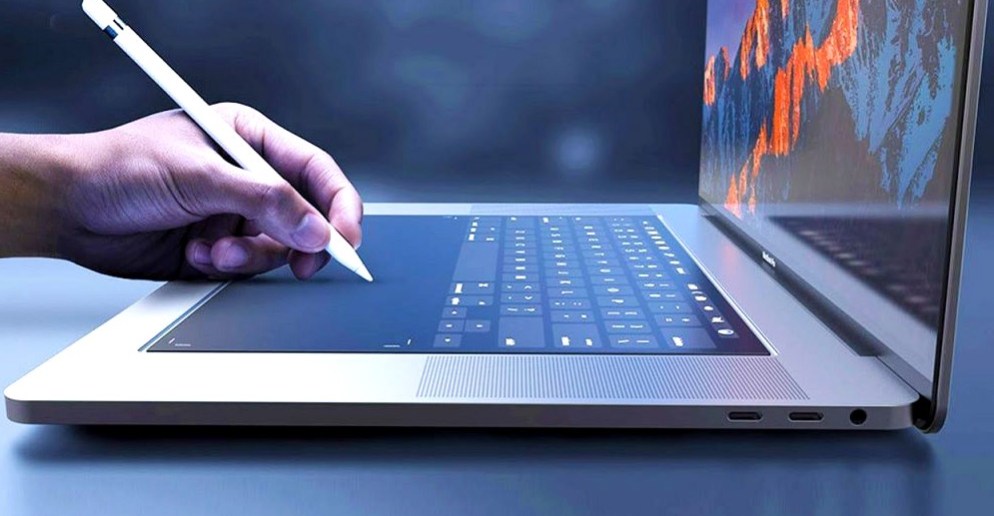
Best Programming laptops to buy in 2021
May 23, 2021, 12:19 p.m.
What is Data Science & How to become a Data Scientist - A complete Guide for Beginners
June 21, 2021, 3:08 p.m.
Linear Regression Using Gradient Descent Python
Aug. 26, 2021, 10:22 a.m.